本文主要讲解在vue项目中如何使用Arcgis Api for javascript的esri/wigets
。
AreaMeasurement2D
更多配置请查看官网:AreaMeasurement2D
1
| require(['esri/widgets/AreaMeasurement2D'], function(AreaMeasurement2D) { });
|
计算并显示地图视图中多边形的面积和周长。这个小部件将为地理坐标系和WebMercator计算面积和周长。
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| <template> <div class="page"> <div id="map"> <div id="topbar" ref="topbar"> <button class="action-button esri-icon-measure-line"></button> </div> </div> </div> </template>
<script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, activeWidget: null, publicPath: process.env.BASE_URL } }, methods: { measure2D (AreaMeasurement2D) { if (this.activeWidget === null) { this.activeWidget = new AreaMeasurement2D({ view: this.view }) this.activeWidget.viewModel.newMeasurement() this.view.ui.add(this.activeWidget, 'top-right') } else { this.view.ui.remove(this.activeWidget) this.activeWidget.destroy() this.activeWidget = null } } }, mounted () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/Map', 'esri/views/MapView', 'esri/widgets/AreaMeasurement2D' ], options) .then(([ArcGISMap, MapView, AreaMeasurement2D]) => { this.map = new ArcGISMap({ basemap: 'hybrid' }) this.view = new MapView({ container: 'map', map: this.map, zoom: 4 }) this.view.ui.add('topbar', 'top-right') this.$refs.topbar.addEventListener('click', () => { this.measure2D(AreaMeasurement2D) }) }) } } </script>
<style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } #topbar { background: #fff; padding: 10px; .action-button { font-size: 16px; background-color: transparent; border: 1px solid #d3d3d3; color: #6e6e6e; height: 32px; width: 32px; text-align: center; box-shadow: 0 0 1px rgba(0, 0, 0, 0.3); } .action-button:hover, .action-button:focus { background: #0079c1; color: #e4e4e4; } .active { background: #0079c1; color: #e4e4e4; } } </style>
|
效果如图:
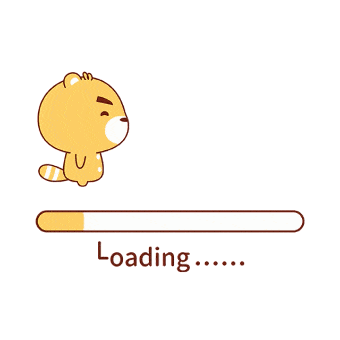
解释:
this.$refs.topbar.addEventListener
使用
不能采用如下这种方式,如果这样做,就相当于立即执行了该函数1
| this.$refs.topbar.addEventListener('click', this.measure2D(AreaMeasurement2D))
|
需要采用这种方式:1 2 3
| this.$refs.topbar.addEventListener('click', () => { this.measure2D(AreaMeasurement2D) })
|
为什么不用@click="measure2D"
?
因为我们需要将AreaMeasurement2D
传递到measure2D
函数中,如果使用@click="measure2D"
就无法传递。
2. activeWidget.viewModel.newMeasurement()
说明
使用这行代码就会跳过AreaMeasurement2D
初始的“新测量”按钮,如果不加这一行,会在右上角生成新测量按钮:如图
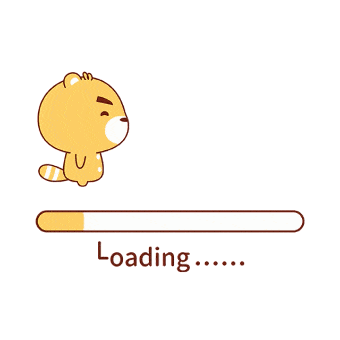
AreaMeasurement3D
更多配置请查看官网:AreaMeasurement3D
1
| require(['esri/widgets/AreaMeasurement3D'], function(AreaMeasurement3D) { });
|
计算并显示多边形的面积和周长。这个小部件可以在场景视图中用来测量多边形的面积和周长。
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100
| <template> <div class="page"> <div id="map"> <div id="topbar" ref="topbar"> <button class="action-button esri-icon-measure-area"></button> </div> </div> </div> </template> <script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, activeWidget: null, publicPath: process.env.BASE_URL } }, methods: { measure2D (AreaMeasurement3D) { if (this.activeWidget === null) { this.activeWidget = new AreaMeasurement3D({ view: this.view }) this.activeWidget.viewModel.newMeasurement() this.view.ui.add(this.activeWidget, 'top-right') } else { this.view.ui.remove(this.activeWidget) this.activeWidget.destroy() this.activeWidget = null } } }, mounted () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/WebScene', 'esri/views/SceneView', 'esri/widgets/AreaMeasurement3D' ], options) .then(([WebScene, SceneView, AreaMeasurement3D]) => { this.map = new WebScene({ portalItem: { id: 'b6c889ff1f684cd7a65301984b80b93d' } }) this.view = new SceneView({ container: 'map', map: this.map }) this.view.ui.add('topbar', 'top-right') this.$refs.topbar.addEventListener('click', () => { this.measure2D(AreaMeasurement3D) }) }) } } </script> <style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } #topbar { background: #fff; padding: 10px; .action-button { font-size: 16px; background-color: transparent; border: 1px solid #d3d3d3; color: #6e6e6e; height: 32px; width: 32px; text-align: center; box-shadow: 0 0 1px rgba(0, 0, 0, 0.3); } .action-button:hover, .action-button:focus { background: #0079c1; color: #e4e4e4; } .active { background: #0079c1; color: #e4e4e4; } } </style>
|
效果如下:
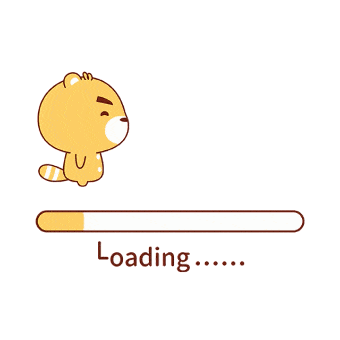
DistanceMeasurement2D
更多配置请查看官网:DistanceMeasurement2D
1
| require(['esri/widgets/DistanceMeasurement2D'], function(DistanceMeasurement2D) { });
|
小部件计算并显示地图视图中两个或多个点之间的距离。这个小部件将计算地理坐标系和WebMercator的地理距离。
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100
| <template> <div class="page"> <div id="map"> <div id="topbar" ref="topbar"> <button class="action-button esri-icon-measure-line"></button> </div> </div> </div> </template> <script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, activeWidget: null, publicPath: process.env.BASE_URL } }, methods: { measure2D (DistanceMeasurement2D) { if (this.activeWidget === null) { this.activeWidget = new DistanceMeasurement2D({ view: this.view }) this.activeWidget.viewModel.newMeasurement() this.view.ui.add(this.activeWidget, 'top-right') } else { this.view.ui.remove(this.activeWidget) this.activeWidget.destroy() this.activeWidget = null } } }, mounted () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/WebMap', 'esri/views/MapView', 'esri/widgets/DistanceMeasurement2D' ], options) .then(([WebMap, MapView, DistanceMeasurement2D]) => { this.map = new WebMap({ portalItem: { id: '990d0191f2574db495c4304a01c3e65b' } }) this.view = new MapView({ container: 'map', map: this.map }) this.view.ui.add('topbar', 'top-right') this.$refs.topbar.addEventListener('click', () => { this.measure2D(DistanceMeasurement2D) }) }) } } </script> <style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } #topbar { background: #fff; padding: 10px; .action-button { font-size: 16px; background-color: transparent; border: 1px solid #d3d3d3; color: #6e6e6e; height: 32px; width: 32px; text-align: center; box-shadow: 0 0 1px rgba(0, 0, 0, 0.3); } .action-button:hover, .action-button:focus { background: #0079c1; color: #e4e4e4; } .active { background: #0079c1; color: #e4e4e4; } } </style>
|
效果如下:
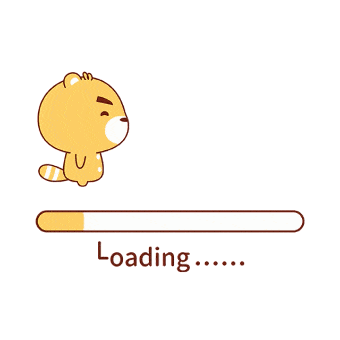
DirectLineMeasurement3D
更多配置请查看官网:DirectLineMeasurement3D
1
| require(['esri/widgets/DirectLineMeasurement3D'], function(DirectLineMeasurement3D) { });
|
部件计算并显示两点之间的三维距离。这个小部件可以在SceneView中用来测量两点之间的垂直、水平和直接距离。
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100
| <template> <div class="page"> <div id="map"> <div id="topbar" ref="topbar"> <button class="action-button esri-icon-measure-line"></button> </div> </div> </div> </template> <script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, activeWidget: null, publicPath: process.env.BASE_URL } }, methods: { measure2D (DirectLineMeasurement3D) { if (this.activeWidget === null) { this.activeWidget = new DirectLineMeasurement3D({ view: this.view }) this.activeWidget.viewModel.newMeasurement() this.view.ui.add(this.activeWidget, 'top-right') } else { this.view.ui.remove(this.activeWidget) this.activeWidget.destroy() this.activeWidget = null } } }, mounted () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/WebScene', 'esri/views/SceneView', 'esri/widgets/DirectLineMeasurement3D' ], options) .then(([WebScene, SceneView, DirectLineMeasurement3D]) => { this.map = new WebScene({ portalItem: { id: 'b6c889ff1f684cd7a65301984b80b93d' } }) this.view = new SceneView({ container: 'map', map: this.map }) this.view.ui.add('topbar', 'top-right') this.$refs.topbar.addEventListener('click', () => { this.measure2D(DirectLineMeasurement3D) }) }) } } </script> <style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } #topbar { background: #fff; padding: 10px; .action-button { font-size: 16px; background-color: transparent; border: 1px solid #d3d3d3; color: #6e6e6e; height: 32px; width: 32px; text-align: center; box-shadow: 0 0 1px rgba(0, 0, 0, 0.3); } .action-button:hover, .action-button:focus { background: #0079c1; color: #e4e4e4; } .active { background: #0079c1; color: #e4e4e4; } } </style>
|
效果如下:
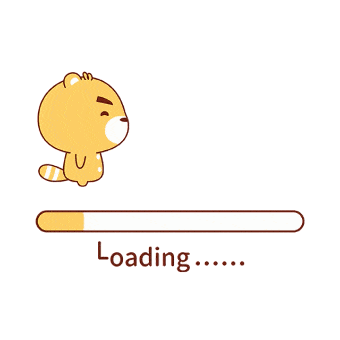
Measurement
更多配置请查看官网:Measurement
1
| require(['esri/widgets/Measurement'], function(Measurement) { });
|
部件计算并显示两点之间的三维距离。这个小部件可以在SceneView中用来测量两点之间的垂直、水平和直接距离。
示例:
官方示例
BasemapGallery
BasemapGallery
小部件显示一个集合,该集合表示来自ArcGIS.com网站或用户定义的一组地图或图像服务。从BasemapGallery中选择新的基础地图时,地图的基础地图图层将被删除,并替换为在库中选择的关联基础地图的基础地图图层。
BasemapGallery
1
| require(['esri/widgets/BasemapGallery'], function(BasemapGallery) { });
|
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
| <template> <div class="page"> <div id="map"> </div> </div> </template> <script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, publicPath: process.env.BASE_URL } }, methods: { renderMap () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/Map', 'esri/views/MapView', 'esri/widgets/BasemapGallery' ], options) .then(([Map, MapView, BasemapGallery]) => { this.map = new Map({ basemap: 'hybrid' }) this.view = new MapView({ map: this.map, container: 'map', zoom: 4, center: [104.051196, 30.573084] }) var basemapGallery = new BasemapGallery({ view: this.view }) this.view.ui.add(basemapGallery, 'top-right') }) } }, mounted () { this.renderMap() } } </script> <style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } </style>
|
效果如下:
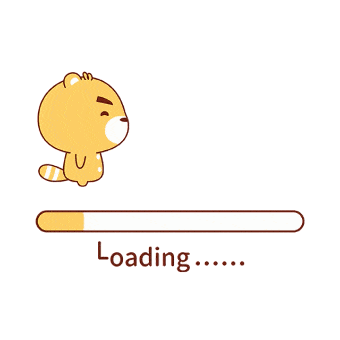
BasemapToggle
BasemapToggle提供了一个小部件,允许最终用户在两个Basemap之间切换。切换的底图设置在视图的map对象内。
BasemapToggle
1
| require(['esri/widgets/BasemapToggle'], function(BasemapToggle) { });
|
示例:
官方示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| <template> <div class="page"> <div id="map"> </div> </div> </template> <script> import esriLoader from 'esri-loader' export default { name: 'Home', data () { return { view: null, map: null, publicPath: process.env.BASE_URL } }, methods: { renderMap () { const options = { url: `${this.publicPath}4.17/init.js`, css: `${this.publicPath}4.17/esri/themes/dark-blue/main.css` } esriLoader.loadModules([ 'esri/Map', 'esri/views/MapView', 'esri/widgets/BasemapToggle' ], options) .then(([Map, MapView, BasemapToggle]) => { this.map = new Map({ basemap: 'hybrid' }) this.view = new MapView({ map: this.map, container: 'map', zoom: 4, center: [104.051196, 30.573084] }) var basemapToggle = new BasemapToggle({ view: this.view, nextBasemap: 'topo-vector' }) this.view.ui.add(basemapToggle, 'bottom-right') }) } }, mounted () { this.renderMap() } } </script> <style lang="scss"> .page{ width: 100%; overflow: hidden; flex: 1 1 auto; position: relative; } #map { width: 100%; height: 100%; } </style>
|
效果如下:
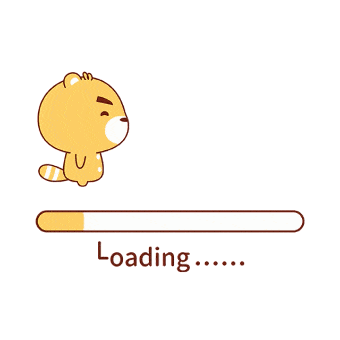
Bookmarks
Bookmarks小部件允许最终用户快速导航到感兴趣的特定区域。它显示书签列表,这些书签通常在WebMap中定义。
Bookmarks
示例:
官方示例
官方示例
官方示例
官方示例
BuildingExplorer
BuildingExplorer小部件用于过滤和浏览BuildingSceneLayers的各个组件。建筑场景是建筑物和内部的复杂数字模型,可以包含数千个分组在子层中的组件。使用此小部件,可以按级别、施工阶段或按规程和类别筛选建筑物。
BuildingExplorer
示例:
官方示例
Compass
Compass小部件指示相对于当前视图旋转或相机航向的北向位置。单击“指南针”小部件将视图旋转为朝北(航向=0)。默认情况下,此小部件将添加到SceneView。
Compass
示例:
官方示例
CoordinateConversion
CoordinateConversion小部件提供了一种将用户光标位置显示为地图坐标或几种常用坐标符号之一的方法。此外,小部件还提供了一种将用户输入坐标转换为点的方法。
CoordinateConversion
示例:
官方示例
官方示例
Daylight
Daylight小部件可用于操纵时间和日期,并在SceneView中切换阴影。更改时间和日期时,太阳和星星的位置会相应地更新,从而更改场景中的照明和阴影。
Daylight
示例:
官方示例
Directions
Directions小部件提供了一种使用ArcGIS Online和自定义网络分析路线服务构建驾驶和步行方向的方法。与RouteTask的工作方式类似,这个小部件生成一条路由,使用指定的网络在多个点之间寻找成本最低的路径。搜索地址时,用于导航的点的位置取决于搜索属性的locationType。
Directions
示例:
官方示例
官方示例
Editor
Editor小部件提供开箱即用的编辑体验,以帮助简化web应用程序中的编辑体验。小部件有两个不同的工作流:CreateWorkflow和UpdateWorkflow。这些工作流允许您在可编辑要素图层中添加要素或编辑和/或删除现有要素。
Editor
示例:
官方示例
官方示例
官方示例
官方示例
官方示例
Expand
Expand小部件充当打开小部件的可单击按钮。
Expand
示例:
官方示例
FeatureForm小部件显示功能的属性。此小部件根据功能的属性以及字段是否允许编辑来呈现输入字段。
FeatureForm
示例:
官方示例
官方示例
官方示例
Fullscreen
Fullscreen提供一个简单的小部件来显示视图或使用整个屏幕的用户定义的HTMLElement。
Fullscreen
示例:
官方示例
Home
Home提供一个简单的小部件,用于将视图切换到其初始视点或先前定义的视点。
Home
示例:
官方示例
LayerList
LayerList小部件提供了一种显示层列表并打开/关闭其可见性的方法。ListItemAPI提供对每个层的属性的访问,允许开发人员配置与层相关的操作,并允许开发人员向与层相关的项添加内容。
LayerList
示例:
官方示例
Legend
Legend小部件显示地图中图层的标签和符号。标签及其相应的符号取决于在层的渲染器中设置的值。图例将仅显示视图中可见的图层和子图层。
Legend
示例:
官方示例
LineOfSight
LineOfSight小部件是一个三维分析工具,允许您在SceneView中执行可见性分析。给定观察者和多个目标点之间的可见性是根据视图中当前显示的内容(包括地面、集成网格和三维对象(如建筑物或树木))计算的。
LineOfSight
示例:
官方示例
Locate
Locate提供一个简单的小部件,将视图动画化到用户的当前位置。
Locate
示例:
官方示例
NavigationToggle
NavigationToggle提供两个简单的按钮来切换SceneView的导航模式。请注意,此小部件仅用于SceneView中的3D鼠标交互。它对触摸导航没有影响,并且不应与地图视图中的二维鼠标交互一起使用。
NavigationToggle
示例:
Popup小部件允许用户查看功能属性中的内容。弹出窗口通过为用户提供一种简单的方式来交互和查看层中的属性,从而增强了web应用程序。它们在向用户传递信息方面起着重要的作用,从而提高了应用程序的讲故事能力。
Popup
示例:
官方示例
Print
Print小部件将应用程序与打印服务连接起来,以允许打印地图。它利用了服务器端、高质量、完整的地图打印功能,使用ArcGIS的ExportWebMap服务,可以配置自定义布局模板。其中一个只显示地图,而另一个提供带有图例等的布局。Print小部件与PrintTask一起工作,PrintTask生成地图的打印机就绪版本。
Print
示例:
官方示例
ScaleBar
ScaleBar小部件在地图上或指定的HTML节点中显示比例尺。小部件遵循各种坐标系,并以公制或非公制值显示单位。公制值根据比例显示公里或米,同样,非公制值根据比例显示英里和英尺。
ScaleBar
示例:
官方示例
ScaleRangeSlider
ScaleRangeSlider小部件允许用户根据指定的缩放范围设置最小和最大缩放。当一个层被提供给小部件时,minScale和maxScale被设置为该层的缩放范围。
ScaleRangeSlider
示例:
Search
Search小部件提供了一种对定位器服务、地图/要素服务要素层和/或表执行搜索操作的方法。如果将定位器与地理编码服务一起使用,则使用findaddress操作,而查询则在要素图层上使用。
Search
示例:
官方示例
Sketch
Sketch小部件提供了一个简单的UI,用于在MapView或SceneView上创建和更新图形。这大大减少了在视图中处理图形所需的代码。它用于存储在其图层属性中的图形。
Sketch
示例:
官方示例
Slice
Slice切片小部件是一个三维分析工具,可以用来显示SceneView中被遮挡的内容。“切片”小部件可以应用于任何层类型,从而可以查看建筑物内部或探索地质表面。
Slice
示例:
官方示例
Slider
Slider一个滑块小部件,可用于过滤数据或从用户处收集数字输入。滑块可以有多个拇指,并提供格式化标签和控制用户输入的功能。
Slider
示例:
官方示例
Swipe
Swipe滑动小部件提供了一个工具来显示地图顶部一个或多个图层的一部分。图层可以垂直或水平滑动,以方便比较两个图层或查看图层下面的内容。
Swipe
示例:
官方示例
TableList
TableList小部件提供了一种显示与地图关联的表列表的方法。它用于要素图层表。
TableList
示例:
官方示例
TimeSlider
TimeSlider小部件简化了应用程序中时态数据的可视化。在将时间滑块添加到应用程序之前,首先应该了解如何配置它以正确显示时态数据。
TimeSlider
示例:
官方示例
Track
Track提供一个简单的按钮,用于在单击时将视图设置为用户位置的动画。视图根据被跟踪设备朝向的方向旋转。
Track
示例:
官方示例
Zoom
Zoom缩放小部件允许用户在视图中放大/缩小。
Zoom
示例: